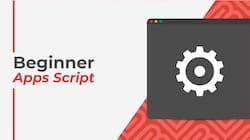
These 10 coding tips will help you develop good practices early in your coding journey.
Learning a programming language is hard. The amount of information feels overwhelming at first. However, by focussing on a few key concepts and techniques, you can make rapid progress.
Use these 10 coding tips to learn Google Apps Script effectively:
1. Make Your Code Understandable
Use plenty of white space and comments in your Apps Script code:
function getData() {
// code here...
}
Don’t worry about trying to make your code concise when you’re learning, better you understand it when you come back to look at it the next day or next week.
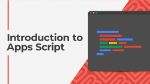
Learn more about Google Apps Script in this free, beginner Introduction To Google Apps Script course
2. Use these keyboard shortcuts when working in the editor
Use these keyboard shortcuts to work more efficiently in the Apps Script editor. These simple shortcuts are SO useful when you’re working with Apps Script that I implore you to take a few minutes today to try them out!
Auto Comment
Auto Comment with:
Ctrl + /
This works on individual lines or blocks of your Apps Script code.
Move code up and down
Move code up and down with:
Alt + Up/Down
If you find yourself wanting to move code around, this is SUPER handy.
Tidy up indentation
Tidy up indentation with:
Tab
Keeping your code properly indented makes it much easier to read and understand. This handy shortcut will help you do that. It’s especially useful if you’ve copied code from somewhere else and the indenting is all higgledy-piggledy.
Bring up the code auto-complete
Bring up the Apps Script code auto-complete with:
Ctrl + Space
(or Ctrl + Alt + Space
on Chromebook)
How many times have you been typing a class or method, made a spelling mistake only to see the helpful auto-complete list disappear? Bring it back with Ctrl + Space (or Ctrl + Alt + Space on Chromebook).
3. Record a macro and look at the code
If you’re not sure how to write something in code, or you’re trying something new, record a Google Sheets Macro for that action and review the code.
The macro tool doesn’t always generate the most concise code, but it will give you helpful clues on how to do certain tasks. You can copy snippets of code and utilize them in your own code.
4. Log Everything with Google script logger
Use the Google script logger Logger.log() method liberally when you’re getting started.
It prints out the values of whatever you “log”, for example the output of a function call. It’s super helpful for you to see what’s going on inside your script at different stages.
You can also add notes like this:
Logger.log("Hey, this function X just got called!");
If you see this in your logs, then you know that function X was called.
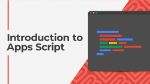
Learn more about Google Apps Script in this free, beginner Introduction To Google Apps Script course
This is probably the most useful tip from these 10 coding tips!
5. Understand These Four Fundamental Concepts
i) Variables
Variables are placeholders for storing data values. You create variables with the var notation and assign values with a single equals sign.
For example, the following expression sets the variable counter to have a value of 0. Anytime you use counter
in your code, it will have the value 0, until you change it.
var counter = 0;
ii) Functions
Functions are blocks of code designed to perform a specific task. A function is run (executed) when something calls it (invokes it).
Functions can be declared (created) with the word function
followed by the function name, which is getData
in the following example:
function getData() {
// code here...
}
The brackets immediately after the function name are required, and are used to hold optional arguments, in a similar way to how functions are used in Google Sheets.
iii) Arrays
Arrays hold multiple values in a single variable, using a square bracket notation. The order of the values is important. Items are accessed by calling on their position in the array. One other thing to note: the array index starts from 0, not 1!
The following expression creates a new array, called fruitsArray
, with three elements in positions 0, 1 and 2.
var fruitsArray = [ "apple", "banana", "pear" ];
iv) Objects
Objects can hold multiple values too, but think of them as properties belonging to the object. They are stored in key/value pairs. For example, here is an object, stored in a variable called book, which has two key/value property pairs:
var book = {
"title": "Apps Script Book",
"author": "Ben Collins"
}
The order of the pairs does not matter when you write out objects. The values are accessed by calling on the key names.
Obviously there’s a lot more to Apps Script than just these four concepts, but understanding Variables, Functions, Arrays and Objects, and how to work with them, will go a long way towards you creating functional Apps Script programs of your own.
6. Understand the Google Sheets Double Array Notation
This is really, really key to using Apps Script to work with Google Sheets. Once you understand the double array notation for Google Sheets data, you open up a huge range of opportunities for extending your Google Sheets work. Spend enough time with this topic, and it’ll become as familiar as the regular A1 notation in Sheets.
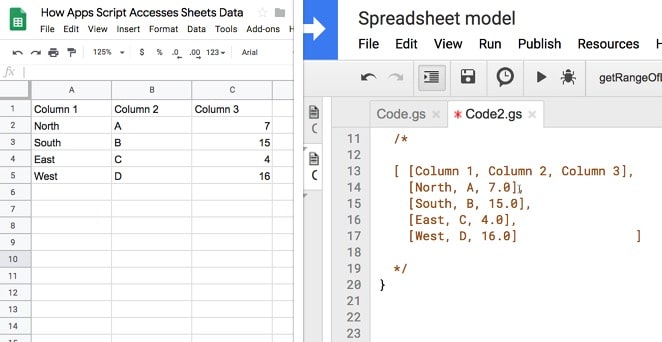
7. Learn basic loops
The For Loop
Start with the basic for
loop to understand how loops work.
It lays bare the mechanics of the loop, showing the starting number, how many times to loop and whether you’re increasing the loop counter or decreasing it.
Logger.log(i);
}
The ForEach Loop
Next up, take some time to learn the more modern looping method: the forEach
loop.
This hides the loop mechanics, which makes for cleaner, more readable code. It’s really easy to work with once you get the hang of it.
Logger.log(item);
});
Basically it grabs all the data from your array and loops over each item in turn. You can do something, by applying a function, to each item during each loop of the array.
8. Understand how Google Sheets <--> Apps Script Transfer Data
Understand how data is passed back and forth between Google Sheets and Apps Script, and how to optimize for that.
Calculations in Google Sheets are done in your browser and are fast. Similarly, calculations done in Apps Script on the Google servers are lightning fast. But passing data back and forth from Sheet to Apps Script or vice versa, oh boy! That’s slow in comparison. (We’re still talking seconds or minutes here, but that’s slow in computing terms.)
To illustrate, here’s a script that retrieves values one cell at a time, performs a calculation in Apps Script and sends the single cell answer back to the Google Sheet. It performs this for one hundred numbers (shown in real time):
Contrast that to the equivalent calculation where the script grabs all one hundred numbers in one, performs the calculations and pastes them back en masse, in one go:
Looks almost instantaneous to the human eye. So much faster!
Here’s another image to summarize this optimization process:
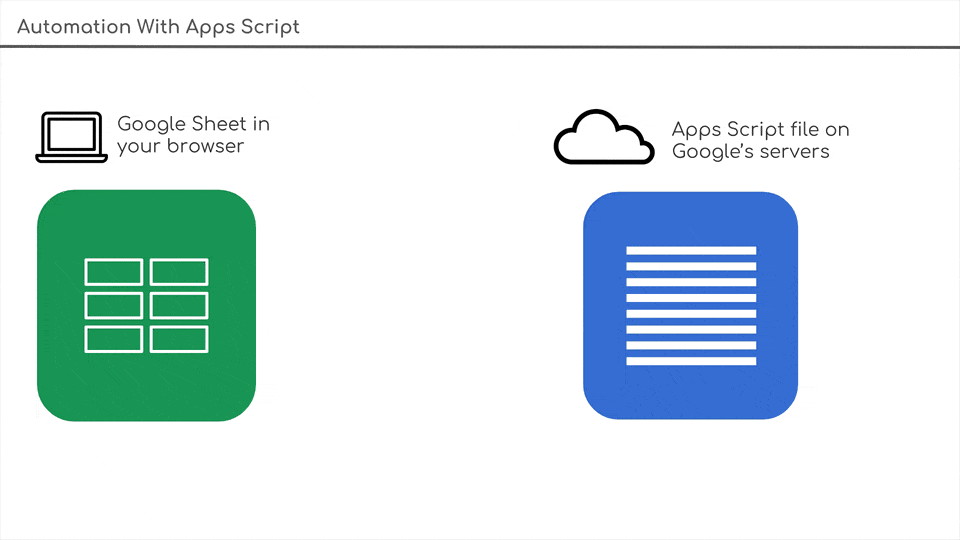
Try to minimize the number of calls you make between your Apps Script and your Google Sheets.
9. Use the Documentation
The Apps Script documentation is your friend.
It might feel overwhelming at first, but persevere and spend time there. Most likely you’ll find something of value to help you solve your current issue.
It’s full of both code examples and a comprehensive reference, so you can look up the precise type of the return value of function X.
10. Ask for help
The final tip of the 10 coding tips is to not be afraid to ask for help when you get stuck.
I always advocate spending time trying to solve your problems yourself, but past a certain point it’s diminishing returns.
Know when to stop banging your head against the wall and try searching for or asking for help in one of these two places:
Google Apps Script Community Group
Stack Overflow Apps Script Tag
Want to learn more coding tips?
Got these 10 coding tips dialed? Want to keep learning. Here are some more resources to try:
Beginner Tutorials
Guide to simple automation with Google Sheets Macros
Macros are small Apps Script programs that the computer records for you. They’re a gentle way to start with Apps Script.
Google Apps Script: A Beginner’s Guide
Online courses
I’ve created two high quality, online courses teaching Apps Script from the ground up. They’re the best way to learn Apps Script in the shortest amount of time.
The first course, Introduction To Google Apps Script, is designed to take you from 0 to 20 and get you started on your Apps Script journey.
The follow-up course, Automation With Apps Script, is designed to take you from 10 to 100 (or wherever you want to go!) and focuses on how to automate workflows in G Suite and connect to external APIs. This course is available for enrollment twice per year, and the next open enrollment is in early 2020.
Hi Ben.
I get the message ‘No logs found. Use Logger API to add logs to your project.’ when I’m trying to follow along to your course.
The official Google documentation is woefully lacking on this and I have searched their Community for help, without success. Do you have any idea why the logger isn’t working for me. It’s supposed to be built in.
Well, after an hour, and starting again from scratch, it has started working! I THINK it might be because the script permissions window popped up in the background but I’m not entirely sure as I thought I had agreed to it already.
Thank you so much! My AdBlocker was preventing My logger pop up to function properly. (it was displaying “please wait” constantly). I disabled it for google Script editor page and now it works as it should. I now that debugging power in my hands!
Ben,
Can you help me with this posting I put on the Docs Editor Group site? I’ve been trying to resolve this for a week and getting no response.
https://support.google.com/docs/thread/19733417?hl=en
I’m sure it’s probably an easy fix, but I’m not sure what I’m doing wrong.
A great introduction to apps script!
Don’t forget to update loops considering the for.. of loop. It covers more cases than for each and is apparently faster
Hi Ben,
i am trying to use app script to get the urls of a sitempa.xml.gz document but i am struggling with the unzipping of the gz file, any suggestions on how i could go about that?
Thanks,
Alessio