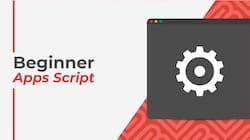
What is Google Apps Script?
Google Apps Script is a cloud-based scripting language for extending the functionality of Google Apps and building lightweight cloud-based applications.
It means you write small programs with Apps Script to extend the standard features of Google Workspace Apps. It’s great for filling in the gaps in your workflows.
With Apps Script, you can do cool stuff like automating repeatable tasks, creating documents, emailing people automatically and connecting your Google Sheets to other services you use.
Writing your first Google Script
In this Google Sheets script tutorial, we’re going to write a script that is bound to our Google Sheet. This is called a container-bound script.
(If you’re looking for more advanced examples and tutorials, check out the full list of Apps Script articles on my homepage.)
Hello World in Google Apps Script
Let’s write our first, extremely basic program, the classic “Hello world” program beloved of computer teaching departments the world over.
Begin by creating a new Google Sheet.
Then click the menu: Extensions > Apps Script
This will open a new tab in your browser, which is the Google Apps Script editor window:
By default, it’ll open with a single Google Script file (code.gs
) and a default code block, myFunction()
:
function myFunction() { }
In the code window, between the curly braces after the function myFunction()
syntax, write the following line of code so you have this in your code window:
function myFunction() { Browser.msgBox("Hello World!"); }
Your code window should now look like this:
Google Apps Script Authorization
Google Scripts have robust security protections to reduce risk from unverified apps, so we go through the authorization workflow when we first authorize our own apps.
When you hit the run button for the first time, you will be prompted to authorize the app to run:
Clicking Review Permissions pops up another window in turn, showing what permissions your app needs to run. In this instance the app wants to view and manage your spreadsheets in Google Drive, so click Allow (otherwise your script won’t be able to interact with your spreadsheet or do anything):
❗️When your first run your apps script, you may see the “app isn’t verified” screen and warnings about whether you want to continue.
In our case, since we are the creator of the app, we know it’s safe so we do want to continue. Furthermore, the apps script projects in this post are not intended to be published publicly for other users, so we don’t need to submit it to Google for review (although if you want to do that, here’s more information).
Click the “Advanced” button in the bottom left of the review permissions pop-up, and then click the “Go to Starter Script Code (unsafe)” at the bottom of the next screen to continue. Then type in the words “Continue” on the next screen, click Next, and finally review the permissions and click “ALLOW”, as shown in this image (showing a different script in the old editor):
More information can be found in this detailed blog post from Google Developer Expert Martin Hawksey.
Running a function in Apps Script
Once you’ve authorized the Google App script, the function will run (or execute).
If anything goes wrong with your code, this is the stage when you’d see a warning message (instead of the yellow message, you’ll get a red box with an error message in it).
Return to your Google Sheet and you should see the output of your program, a message box popup with the classic “Hello world!” message:
Click on Ok to dismiss.
Great job! You’ve now written your first apps script program.
Rename functions in Google Apps Script
We should rename our function to something more meaningful.
At present, it’s called myFunction which is the default, generic name generated by Google. Every time I want to call this function (i.e. run it to do something) I would write myFunction()
. This isn’t very descriptive, so let’s rename it to helloWorld()
, which gives us some context.
So change your code in line 1 from this:
function myFunction() { Browser.msgBox("Hello World!"); }
to this:
function helloWorld() { Browser.msgBox("Hello World!"); }
Note, it’s convention in Apps Script to use the CamelCase naming convention, starting with a lowercase letter. Hence, we name our function helloWorld
, with a lowercase h at the start of hello and an uppercase W at the start of World.
Adding a custom menu in Google Apps Script
In its current form, our program is pretty useless for many reasons, not least because we can only run it from the script editor window and not from our spreadsheet.
Let’s fix that by adding a custom menu to the menu bar of our spreadsheet so a user can run the script within the spreadsheet without needing to open up the editor window.
This is actually surprisingly easy to do, requiring only a few lines of code. Add the following 6 lines of code into the editor window, above the helloWorld()
function we created above, as shown here:
function onOpen() { const ui = SpreadsheetApp.getUi(); ui.createMenu('My Custom Menu') .addItem('Say Hello', 'helloWorld') .addToUi(); } function helloWorld() { Browser.msgBox("Hello World!"); }
If you look back at your spreadsheet tab in the browser now, nothing will have changed. You won’t have the custom menu there yet. We need to re-open our spreadsheet (refresh it) or run our onOpen()
script first, for the menu to show up.
To run onOpen()
from the editor window, first select then run the onOpen function as shown in this image:
Now, when you return to your spreadsheet you’ll see a new menu on the right side of the Help option, called My Custom Menu. Click on it and it’ll open up to show a choice to run your Hello World program:
Run functions from buttons in Google Sheets
An alternative way to run Google Scripts from your Sheets is to bind the function to a button in your Sheet.
For example, here’s an invoice template Sheet with a RESET button to clear out the contents:
For more information on how to do this, have a look at this post: Add A Google Sheets Button To Run Scripts
Google Apps Script Examples
Macros in Google Sheets
Another great way to get started with Google Scripts is by using Macros. Macros are small programs in your Google Sheets that you record so that you can re-use them (for example applying standard formatting to a table). They use Apps Script under the hood so it’s a great way to get started.
Read more: The Complete Guide to Simple Automation using Google Sheets Macros
Custom function using Google Apps Script
Let’s create a custom function with Apps Script, and also demonstrate the use of the Maps Service. We’ll be creating a small custom function that calculates the driving distance between two points, based on Google Maps Service driving estimates.
The goal is to be able to have two place-names in our spreadsheet, and type the new function in a new cell to get the distance, as follows:
The solution should be:
Copy the following code into the Apps Script editor window and save. First time, you’ll need to run the script once from the editor window and click “Allow” to ensure the script can interact with your spreadsheet.
function distanceBetweenPoints(start_point, end_point) { // get the directions const directions = Maps.newDirectionFinder() .setOrigin(start_point) .setDestination(end_point) .setMode(Maps.DirectionFinder.Mode.DRIVING) .getDirections(); // get the first route and return the distance const route = directions.routes[0]; const distance = route.legs[0].distance.text; return distance; }
Saving data with Google Apps Script
Let’s take a look at another simple use case for this Google Sheets Apps Script tutorial.
Suppose I want to save copy of some data at periodic intervals, like so:
In this script, I’ve created a custom menu to run my main function. The main function, saveData()
, copies the top row of my spreadsheet (the live data) and pastes it to the next blank line below my current data range with the new timestamp, thereby “saving” a snapshot in time.
The code for this example is:
// custom menu function function onOpen() { const ui = SpreadsheetApp.getUi(); ui.createMenu('Custom Menu') .addItem('Save Data','saveData') .addToUi(); } // function to save data function saveData() { const ss = SpreadsheetApp.getActiveSpreadsheet(); const sheet = ss.getSheets()[0]; const url = sheet.getRange('Sheet1!A1').getValue(); const follower_count = sheet.getRange('Sheet1!B1').getValue(); const date = sheet.getRange('Sheet1!C1').getValue(); sheet.appendRow([url,follower_count,date]); }
See this post: How To Save Data In Google Sheets With Timestamps Using Apps Script, for a step-by-step guide to create and run this script.
Google Apps Script example in Google Docs
Google Apps Script is by no means confined to Sheets only and can be accessed from other Google Workspace tools.
Here’s a quick example in Google Docs, showing a script that inserts a specific symbol wherever your cursor is:
We do this using Google App Scripts as follows:
1. Create a new Google Doc
2. Open script editor from the menu: Extensions > Apps Script
3. In the newly opened Script tab, remove all of the boilerplate code (the “myFunction” code block)
4. Copy in the following code:
// code to add the custom menu function onOpen() { const ui = DocumentApp.getUi(); ui.createMenu('My Custom Menu') .addItem('Insert Symbol', 'insertSymbol') .addToUi(); } // code to insert the symbol function insertSymbol() { // add symbol at the cursor position const cursor = DocumentApp.getActiveDocument().getCursor(); cursor.insertText('§§'); }
5. You can change the special character in this line
cursor.insertText('§§');
to whatever you want it to be, e.g.
cursor.insertText('( ͡° ͜ʖ ͡°)');
6. Click Save and give your script project a name (doesn’t affect the running so call it what you want e.g. Insert Symbol)
7. Run the script for the first time by clicking on the menu: Run > onOpen
8. Google will recognize the script is not yet authorized and ask you if you want to continue. Click Continue
9. Since this the first run of the script, Google Docs asks you to authorize the script (I called my script “test” which you can see below):
10. Click Allow
11. Return to your Google Doc now.
12. You’ll have a new menu option, so click on it:
My Custom Menu > Insert Symbol
13. Click on Insert Symbol and you should see the symbol inserted wherever your cursor is.
Google Apps Script Tip: Use the Logger class
Use the Logger class to output text messages to the log files, to help debug code.
The log files are shown automatically after the program has finished running, or by going to the Executions menu in the left sidebar menu options (the fourth symbol, under the clock symbol).
The syntax in its most basic form is Logger.log(something in here)
. This records the value(s) of variable(s) at different steps of your program.
For example, add this script to a code file your editor window:
function logTimeRightNow() { const timestamp = new Date(); Logger.log(timestamp); }
Run the script in the editor window and you should see:
Real world examples from my own work
I’ve only scratched the surface of what’s possible using G.A.S. to extend the Google Apps experience.
Here are a couple of interesting projects I’ve worked on:
1) A Sheets/web-app consisting of a custom web form that feeds data into a Google Sheet (including uploading images to Drive and showing thumbnails in the spreadsheet), then creates a PDF copy of the data in the spreadsheet and automatically emails it to the users. And with all the data in a master Google Sheet, it’s possible to perform data analysis, build dashboards showing data in real-time and share/collaborate with other users.
2) A dashboard that connects to a Google Analytics account, pulls in social media data, checks the website status and emails a summary screenshot as a PDF at the end of each day.
3) A marking template that can send scores/feedback to students via email and Slack, with a single click from within Google Sheets. Read more in this article: Save time with this custom Google Sheets, Slack & Email integration
My own journey into Google Apps Script
My friend Julian, from Measure School, interviewed me in May 2017 about my journey into Apps Script and my thoughts on getting started:
Google Apps Script Resources
For further reading, I’ve created this list of resources for information and inspiration:
Course
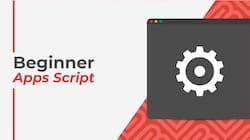
Documentation
Google Workspace Developers Blog
Communities
Elsewhere On The Internet
A huge big up-to-date list of Apps Script resources hosted on GitHub.
For general Javascript questions, I recommend this JavaScript tutorial page from W3 Schools when you’re starting out.
When you’re more comfortable with Javascript basics, then I recommend the comprehensive JavaScript documentation from Mozilla.
Imagination and patience to learn are the only limits to what you can do and where you can go with GAS. I hope you feel inspired to try extending your Sheets and Docs and automate those boring, repetitive tasks!
I couldn’t resist commenting. Exceptionally well
written!
Thank you!
Hey Ben,
I am facing error in code for calculating distance between 2 points
Cannot read property “legs” from undefined. (line 11, file “Code”)
Can you please help
@Rishu, were you able to fix it? I’m facing the same problem. Strangely enough it doesn’t happen all the time, script works several times and then suddenly starts giving the error. I have searched the web and it seems to be a recurring problem with no definite answer. Any help will be appreciated.
Regards
We need to have Google Cloud credential to enable the Maps API in order for us to continue using the API
Me too for some reason
Hi Ben,
How far did you get with project number 1 above. Kindly share a tutorial.
Hi Ben – Awesome work! Like Cromwel, I would love to hear more on your project#1. Thank you for sharing.
Hi is there print preview command in Google sheet.. by getrange(‘a:b’) I want to print a certain range
Hi, Im writing some code in google script, but when I open de file, my script doesn’t work until the first minute i Think, its like there is a delay before the script really respond to my calls on buttons, do you know why is this happening?
Hi Armando,
Hmm, there shouldn’t be any substantial delay, certainly not a minute. The script could be affected by your internet connection speed, but I wouldn’t expect to see such a big delay, especially if other web services load normally.
Inefficient code, i.e. lots of calls to/from the Google Sheets or other Google services, can make a script run slowly, so it’s best practice to minimize the number of calls required. More reading here at the official Apps Script docs: https://developers.google.com/apps-script/best_practices
I suggest sharing your question/code on the Apps Script forum here: https://plus.google.com/u/0/communities/102471985047225101769 where there’s a community of experts who can suggest specific fixes.
Hope that helps.
Ben
hi, i want to track a user who copy, cut, or delete the more then 10 cells at once, please provide me a code or a way how can i do it
Hi, Ben,
Thanks for very well written article that even a beginner like myself could understand! What resources/training courses/tutorials on Google App scripts you would advise to a person that have no previous knowledge of programming.
Thanks,
Sat
Hi Sat, there’s a list of resources at the end of this post which is where I’d start. Then try creating your own scripts and googling for answers when things don’t work. The forums are very helpful at answering specific questions.
Cheers,
Ben
Hi, I’m french, so excuse me for my approximate English …
I’ve tested your “Custom function/maps example” paragraph, but I’ve an error !
When I validate the formula in spreadsheet it says: TypeError: Unable to read the “legs” property from undefined. (Line 12).
Can you help me ??
Thx
Here’s an answer I found if your still having trouble from http://www.gadgetsappshacks.com/2014/02/calculating-distance-between-two.html:
———
This happens if the cell being referenced by the function (e.g. cell A8 in the pubic spreadsheet I provided) is made an invalid location (e.g. “XASD*&^”). So perhaps one of our locations isn’t something that Google Maps can uniquely identify as a location. Try cut/pasting it into maps.google.com to see how it responds.
———-
My script was doing the same thing until I opened up Google Maps
Thanks for sharing your solution Sebastian!
I found that if the location is one of several that would be returned from a Maps search, an error is displayed. No real surprise there. You have to specify enough detail to make it unique. Here in the UK I was searching for ‘Dover’, but until I put ‘Dover, Kent’ the distance was not displayed.
Interesting, thanks for sharing Gary. I’ve never taken this function beyond this very basic example for illustration, but clearly you could improve the error handling and give this kind of feedback to a user. Cheers!
Hi Ben,
this article is really interesting.
I found the dashboard that connects to a Google Analytics account, pulls in social media data, checks the website status and emails really amazing and extremely useful. Do you plan to share it or to make a tutorial on how to built a dashboard like that?
Thanks,
Stefano
Hey Stefano,
That dashboard is covered in depth in the online dashboard course I’m launching….next week (20th Feb)! I walk through how to create it step-by-step, including the apps scripting.
P.S. There’ll be a special offer for newsletter subscribers next week (sign up form at the end of this page here).
Cheers,
Ben
Very interesting. Am in dire need to learn how to develop a mobile app for e-learning/assessment. However, I don’t have knowledge of programming. I will appreciate if I can get a step by step guide on programming. Thanks. Augustine
Very good information. Lucky me I found your blog by accident (stumbleupon).
I have saved it for later!
I get an error: Cannot call Browser.msgBox() from this context; have you tried Logger.log() instead? (line 2, file “Code”) when I try to run the Hello World script. Failing at the first attempt does not feel good. Do you know what the problem is?
Hmm, not sure. Did you get the Authorization Required popup? What’s the exact error message you’re getting? The popup will show up in your Sheet browser tab, not the code editor tab, so you need to return to that one to the Hello World message.
I did not get the authorisation pop up when I ran the script. However, i have tried it today and hit the debug icon, instead of run. This DID produce the authorisation pop up, and the function has run fine. Curious behaviour!
Yes, that is strange! Great it’s working for you now 🙂
I also encountered this error. My issue is that I created my script in Google Apps Script, so my script wasn’t directly tied to any Google Sheet. To fix this, I went to the Google Sheet in which I wanted this code to run, clicked on the Tools dropdown menu, and clicked on “Script editor”. This took me to a new Google Apps Script project that was directly tied to that Google Sheet. I was able to run this code successfully by following these steps.
Very useful info..
Had one weird problem when I tried the first UI example..
When I created the menu as you did
e.g.
var ui = SpreadsheetApp.getUi();
ui.createMenu(‘My Custom Menu’)
.addItem(‘Say Hello’, ‘helloWorld’)
.addToUi();
I got a “.createMenu undefined” error…
didn’t shake the error message until I rewrote to
var ui = SpreadsheetApp.getUi().createMenu(‘MyMenu’)
.addItem(‘Hello There’, ‘helloWorld’)
.addToUi();
and it all worked fine.
Have no idea what the difference is.
I’ve had this kind of thing happen before in app script though…
in particular when I was testing for results less than a particular variable using var1 0 and everything worked OK.
so it’s good to teach multiple ways of doing the same thing.
Great Post
Thanks a lot it helped me a lot
I am also going to share it to my friends and over my social media.
Also,
Hackr.io is a great platform to find and share the best tutorials and they have a specific page for Google apps script
This might be useful to your readers: https://hackr.io/tutorials/learn-google-apps-scripts
Hi,
We have a container bound script and would like to password protect the Script Editor.Is there any way to do this or a workaround available to ensure that only the developer can access the Script Editor?
I’m looking for the answer too! and I can not find it after 3 years. Is there any way?
This is awesome – Thanks!
I’m running in to an error with the Map distance problem.
It initially worked, and then when I tried it again with new values, it stated:
“TypeError: Cannot read property “legs” from undefined.”
Even when I put the old values back in, it would no longer work.
Any suggestions?
Hey Mike,
Have a look at this comment thread further up the post: https://www.benlcollins.com/spreadsheets/starting-gas/#comment-17176
It’s most likely caused by ambiguity in the leg, which this basic function isn’t able to deal with.
Cheers,
Ben
Thanks for the quick reply!
The strange thing is that even the two points that initially worked and returned the distance, also stopped working after I tried other destinations. It only worked the first time and then everything seemed to break.
What a lovely article. I’m just starting to explore all the possibilities of Google App Scripting. I don’t have any main goal yet, so I’m just fiddling around a bit.
For starters I’d like to know whatever is possible with an app inside a google site, and I didn’t find any documentation on how to implement custom UI controls on the properties on the embedded app.
To clarify: I add a script in my google site, then on a page I add a “Google Script App”, and add that script I just made. Then for that added ‘widget’, you can click on the edit button. I’d like to know how to add my custom settings on that page.
I know it’s possible, as other existing widgets can do this too.
Any help on this one?
Hey Steven,
I’ve never used Google Sites, so I’m not sure the answer to your question. I’d suggest posting your question to the Google Apps Script forum where I’m sure someone will have some input: https://plus.google.com/u/0/communities/102471985047225101769
Cheers,
Ben
I am new to scripting. I have 12 functions in a single script. I cannot find a suitable answer to this question:
How can I link my scripts so that all 12 run sequentially?
Thank you very much!
Hey Brian,
I can understand your frustration, I was there once too 🙂
You can call your 12 functions inside another, sort of master execution function, if you will, like this:
function runFuncs() {
// put your 12 functions in here...
func1();
func2();
// etc...
}
function func1() {
Logger.log("Hello!")
}
function func2() {
Logger.log("Goodbye!");
}
where func1 and func2 would be the first two of your 12 functions. When you run runFuncs(), it will execute those functions inside of it, func1 and func2 in this case, but could be all your 12.
Hope that helps!
Ben
I am a new user to google sheets and I love working with excel automation and advance excel. I searched about google sheet programming, and your this article become very beneficial to me.
Great work!
Thanks Abdul!
Hey Ben, I have a real beginner’s question.
How do I get the “suggestion screen” on screen when I’m typing code. I have a Chromebook.
Thanks for your answer.
Gert-Jan
Hi Ben, I am using getcharts() to retrieve and save a manually produced chart in google sheets. I keep getting a google server error ‘We’re sorry, a server error occurred. Please wait a bit and try again. (line 4, file “Code”)’. The code is below – can you please help me with what I am doing wrong? Is it because the chart was not created with GAS in the first place?
function myFunction()
{ var spreadsheet1=SpreadsheetApp.getActive();
var sheet = spreadsheet1.getSheets()[0]; // grabs active sheet
var chartvarun = sheet.getCharts(); // grabs the first chart on the sheet
var file = DriveApp.createFile(chart.getBlob()); // saves it as a file
}
@Varun – were you able to solve this? I am getting the same error. Strangely, I am using the identical code on two sheets and only get an error on one.
@Varun or Ben – did this problem ever get resolved? I’m having the same issue and can’t find the solution online. Thanks!
Hi Ben,
I am a junior programmer and my company has asked me to do some google docs work that I have never touched before. Since we are a small company I would love to hear your input…
Can you advise how I would export cvs data from an excel sheet/url to a google doc? I need it automated to occur once a week.
Thanks!
Serena
I have search for a language refernece card for keywords, srtuctures and I could not find yet but some fragments. Any idea where I could find a refcard, a cheat card, thank you
Hello i tried to code the example but i keep gettign this error:
Cannot call Browser.msgBox() from this context; have you tried Logger.log() instead? (line 2, file “Code”)
—————————————————————————-
function myFunction() {
Browser.msgBox(“hello”);
}
I get the same error as Abraham Felix: ‘Cannot call Browser.msgBox() from this context; have you tried Logger.log() instead? (line 2, file “Code”)’.
The Authorization window DID pop up, and I even got an email from Google saying “HelloWorld was granted access to your Google account”, but I still get the ‘Cannot call Browser.msgBox’ error.
As someone pointed out above, getting an error in “Hello World” does not feel encouraging.
Hey Abraham/Kevin,
Are you running this script from the code editor of a Google Sheet? The browser class is only available to Google Sheets, not Docs, Sites, Forms etc.
I just tested it again for me and it works, so not sure why it’s not for you.
Alternative first script: You can change the code to:
function myFunction() {
Logger.log("Hello World!");
}
and then go to the menu View > Logs in your script editor window to see the message.
Cheers,
Ben
how do you do a if then statement
I was reading your post and I really enjoyed it. One quick question. Is there any way to overwrite the same file on Google Drive? I have been reading about it, but everybody mentions that Google uses IDs and that’s why is not an easy way to overwrite a file.
Thanks for your post.
I just found this post and I am so excited to use this for my students. I usually ran multiple mail merges within Word but this is going to save me time since my gradebook is in Google!! Do you have any more articles specifically for teachers?
At the moment, just this one specifically for teachers: https://www.benlcollins.com/spreadsheets/marking-template/
Maybe some in 2019 too!
Your famous dude! I just stumbled across this article – https://blog.markgrowth.com/this-google-spreadsheets-guru-makes-4-000-a-month-on-online-courses-bcda90ddd9e1 – and at the end I thought “hang-on, that name rings a bell. It’s that Ben Collins. Glad to hear the business is going well. I’ve been migrating up towards the GSheet UI rather than being down of the depths of “server-side” Apps Script, and been really enjoying clients with more business/systems analysis and getting GSheets working for them, so I’ll be spending some time on your site learning a bit more about GSheet formulas. Great site by the way. And thanks again for the link 🙂
Hey Andrew,
Haha
hello folks,
where can i found a place with the listed functions of google script?
for now i just wanted one that activated my already saved filter views.
Absolutely awesome, Ben. I know I am late to game vs. the original date of this post, but I am using automation more and more in my worksheets and appreciate the extra time you take explaining how GAS comes together. I aspire to one day do the kind of work reflected in that dashboard! ::drool::
I know this is late for me to comment now or whatever, but for some reason when I get on a project, where the script is supposed to be is blank. Tools and everything is there but the place for me to write scripts are blank and I can’t do anything on it.
Great example, I just wish it worked. There must have been a change since it was posted.
I get the following error “Cannot call SpreadsheetApp.getUi() from this context. (line 2, file “GetCode”)”
Anyone know what to do to fix this? I just want to write something to my spreadsheet ;-(
I had similar problems until I realised it was case sensitive.
Hello! Amazing tutorial. I am trying to run the script for List Growth History, and am getting the following error “TypeError: Cannot call method “forEach” of undefined.” What does this mean and how can I fix it?
Thank you so much!
Best,
Emily
Hey, thanks for the article. searched long google apps script guide. Finally found this blog helpful.
Thanks
Got this article very helpful Ben.
Please help me creating a script for google sheet. the details is;
“Google script to activate the cell on button click. Button activates the cell in which it is placed.”
I have a google sheet in which there is one button in one cell of each row. Each button calls a macro to insert a row below the active cell. The problem is that when I click a button it inserts the row below the active cell. Actually I want that when a particular button is clicked, that particular cell gets activated in which that button is placed. If it happens, a new row will be inserted only below the clicked button not below the active cell somewhere else.
Ben. it will be helpful if you could provide solution. Thanks in Advance.
Hi Ben, I’ve been following your class on Google Sheet Functions and the video tutorials are quite self explanatory — like it so much. What kind of screen casting software you’re using for those videos… Tq!
Thanks! I’m using Screenflow to record, Handbrake to shrink file size.
Great resource, thank you for doing this.
Thanks, John!
Good one Ben. Thanks for the article.
Hi!
How do I create a button that I click on will save it to a specific location and give it a name and date Thank you!
Hey, I’m trying to design a script for when you present the slideshow, it changes a cursor to a specific picture you want with a hotspot (the pixel where the cursor clicks).
Any clue on how to do this?
Hi Ben,
Do you have a tutorial or the codes for the first Real World sheet/web-app example which you mentioned above?
Thank You Ben! Content that is easily digestible for beginners!
When we open the Script Editor from within a Google document for the first time, it automatically creates a new “Untitled project” for us. Let’s change that to something a bit more descriptive, like “Create Headers.
I have a question !
If I am using Map Service within GoogleSheet using Script Editor for calculating Distance b/w two locations , am I gonna pay for it to google or its free ?
I can’t even get “Hello World” to print… it just says “Running Function myfunction… Cancel Dismiss” and never progresses beyond that. I did authorize it as described in the article… it seems so simple yet I can’t even get this simple function to work. Any ideas?
if column E has “get fish” then the email should send, – is this part correct? : –
if (monthSales = “get fish”){
the full code is below: –
function CheckSales() {
// Fetch the monthly sales
var monthSalesRange = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(“myfish”).getRange(“E1:E1000”);
var monthSales = monthSalesRange.getValue();
// Check totals sales
if (monthSales = “get fish”){
// Fetch the email address
var emailRange = SpreadsheetApp.getActiveSpreadsheet().getSheetByName(“Sheet1”).getRange(“B2”);
var emailAddress = emailRange.getValues();
// Send Alert Email.
var message = ‘Get Fish ‘
var subject = ‘Get Fish’;
MailApp.sendEmail(emailAddress, subject, message);
}
}
Great guide!!
I do have a question… We are currently converting from Microsoft Office to Google Suite. We create a TON of documents and have to share them within our organization and outside the organization. Is there a way, when writing script, to have it not ask for authorization to run the script? We have over 700 people that may use these documents and it would be time consuming to have to do this for each file. Most of our documents have script of some kind. Thank you!
Nice information on app script for beginners
You lost me at step one: How do I get to this? “Then click the menu Tools > ” I’m using Chrome I made a new tab… then?
Allen – It’s the Tools menu within your Google Sheet.
Cheers,
Ben
Amazing article to read!
I’m wondering, what are the other functionalities of this Google App Script? Can I build a web-app using GAS? It seems that most people don’t even know if this program exists. Or I’m the only one who should have known this earlier?
Thank you in advance, Ben!
Your website is very beautiful, I like the theme and the style you’re using:)
Good stuff
Hi Ben,
I have tried to practise your first example “Hello World” in Google sheet (my browser using is Google Chrome).
But when I run the script, it shows the ReferenceError: Browser is not defined (line 2, file “Code”). I also tried with ‘logger.log’ (instead of ‘browser.msg’) but the same error.
Could you please let me know how to fix that?
Much appreciated.
Duc
Is it the space in the name “My custom menu”
Nice
This is the best guide on Google Apps Script. After read this article, i can solve my error and run my project on Google Apps Script. I will share this article with my friends. It will be very helpful to all readers. Good job!
Thanks, James!
Hey Ben!
I am enrolled in two of your great courses and I have been learning a lot! I am a beginning into Apps Scripts and I have a question in a problem where I am stuck.
What I am doing with the code below is copying and paste the formula from one cell to the next cell to the right. I am repeating this process in 30 rows. However, I need to tell the code to skip copying and pasting the formula if the row is filtered or hidden and I am unable to make it work.
function fillFormulaDown() {
const sheet = SpreadsheetApp.getActiveSheet();
let currentCell = sheet.getActiveCell();
if(currentCell.isRowHiddenByFilter != true){
while (currentCell.getValue()) {
let formula = currentCell.getFormulaR1C1();
if (formula) {
currentCell.offset(0, 1).setFormulaR1C1(formula);
}
currentCell = currentCell.offset(1, 0);
}
}
}
any inputs would be appreciated.
Hi
I want to link 3 different row status with a fourth one which is the master status for all the 3 status. I have used this following code but it’s only linking with one row and not with the other 2
=ifs(D3=”YTS”,”Wireframe YTS”,D3=”WIP”,”Wireframe WIP”,D3=”RTK”,”Wireframe RTK”,D3=”Review”,”Wireframe Review”,D3=”Correction”,”Wireframe Correction”,D3=”Closed”,”Wireframe Closed”,H3=”YTS”,”Storyboard YTS”,H3=”WIP”,”Storyboard WIP”,H3=”RTK”,”Storyboard RTK”,H3=”Review”,”Storyboard Review”,H3=”Correction”,”Storyboard Correction”,H3=”Closed”,”Storyboard Closed”,L3=”YTS”,”FCD YTS”,L3=”WIP”,”FCD WIP”,L3=”RTK”,”FCD RTK”,L3=”Review”,”FCD Review”,L3=”Correction”,”FCD Correction”,L3=”Closed”,”FCD Closed”)
Excellent !! Easy to understand … Thank you so much !!
Hi Ben,
You are awesome. I have a question to you. I have created a script to get all filename and URL using DriveApp and put the same in spreadsheet but after 1800 seconds (30 minutes) script has stopped its execution with timeout error.
How to handle this if script will take more time in execution what to do to get the required results ?
Pls help
Thanks in advance
Is there any way I can hide top bar default options like file, help etc?
You are a life Saver, Ben. Just weeks ago, I discovered the awesome potential of Google AppScript, and just now I’m on the lookout on how to learn the code, and by far this is the best intro out there. I am specially fond of your mention of Google Docs, because now I have new things to try there.
Thank you so much, Ben.
You’re welcome! Good luck with your coding journey, Mark!
After this article, check out my free Apps Script course: Intro to Apps Script
Congratulations, Ben !
Great materials as your are rarely seen on the Web.
Thanks, Hilton!
This looks great! I want to start making a container-bound script inside a sheet, but I cant’t find the “script editor” in the “tools” menu… Am I missing something? Probably a very noobish beginners question: 😀
Hi Raphael,
It’s moved to the Extensions menu now! Sorry about that. They changed it a couple of months ago and this article needs to be updated. Doing that next!
Cheers,
Ben
Hi, I can get to AppsScript under extensions and open the editor there, but in Google Sheets, under “Tools” there is no “Script Editor” option. Is there something I have to install first?
I read that if you are using an organizations Google Suite, it has the ability to disable the “Script Editor” function, but I don’t know if that applies. I am using a company computer/browser, but the actual Google account is my own. Any idea what is going on?
Hi Tara,
No, you’ve found it. They recently moved the Apps Script menu location from Tools to Extensions. Unfortunately, some of my posts are now out-of-date. I will update this one now.
Cheers,
Ben
I get script funcion not found on my Adding a custom menu in Google Apps Script attempt. Checkit over and over – any clues?
I just started the app script in google excel. But when I am trying to update the existing value then it is not updating. My code is :
function updateData() {
var SPREADSHEET_NAME = “Data”;
var SEARCH_COL_IDX = 0;
var RETURN_COL_IDX = 0;
var ss = SpreadsheetApp.getActiveSpreadsheet();
var formSS = ss.getSheetByName(“Form”); //Form Sheet
var datasheet = ss.getSheetByName(“Data”); //Data Sheet
var str = formSS.getRange(“B5”).getValue();
var values = ss.getSheetByName(SPREADSHEET_NAME).getDataRange().getValues();
for (var i = 0; i < values.length; i++) {
var row = values[i];
if (row[SEARCH_COL_IDX] == str) {
var INT_R = i+1
var values1 = [[formSS.getRange("B5").setValue(row[0]),
formSS.getRange("B7").setValue(row[1]) ,
formSS.getRange("E5").setValue(row[2]) ,
formSS.getRange("E7").setValue(row[3]) ,
formSS.getRange("H5").setValue(row[4]) ,
formSS.getRange("H7").setValue(row[5]) ,
formSS.getRange("B9").setValue(row[6]),
formSS.getRange("E9").setValue(row[7]) ,
formSS.getRange("H9").setValue(row[8]) ,
formSS.getRange("H11").setValue(row[9]) ,
formSS.getRange("H13").setValue(row[10]),
formSS.getRange("B11").setValue(row[11]),
formSS.getRange("D11").setValue(row[12]),
formSS.getRange("B12").setValue(row[13]),
formSS.getRange("D12").setValue(row[14]),
formSS.getRange("B13").setValue(row[15]),
formSS.getRange("D13").setValue(row[16]),
formSS.getRange("B14").setValue(row[17]),
formSS.getRange("D14").setValue(row[18]),
formSS.getRange("B15").setValue(row[19]) ,
formSS.getRange("D15").setValue(row[20]),
formSS.getRange("B16").setValue(row[21]),
formSS.getRange("D16").setValue(row[22]) ]];
datasheet.getRange(INT_R, 1, 1, 23).setValues(values1);
SpreadsheetApp.getUi().alert(' "Data Updated "');
return row[RETURN_COL_IDX];
}
}
}
It is updating the value as "Range".
Please help to rectify this issue
Do you know if it’s possible to write a Google script where you can upload a CSV file of addresses you’ve SENT to, and then have the script filter that list and give you a new CSV file that only includes the addresses that haven’t replied?
Hi,
I need to run 3 scripts for same sheet simultaneously in Google app script. can you please help me?
This is interesting to me as a small-time researcher. It seems like a tool for hobbyists and people who are interested in improving their productivity, possibly as small business owners, as opposed to being used for deployment. I wonder how many people have actually used google apps scripts. I would be interested in the actual statistics. I just started by creating some simple scripts to organize my debts/credits and to send emails. I think it’s a fun tool to use and I think that it has a very high potential for scripting.
I need help.
I was handed an app created by app script. Apart from the fact that I don’t understand App script how can I add it online on our domain so that it can be accessed any where.
I want the app to be connected to mysql database, is it possible?
How do I convert a number into text using Apps Script?
Apps Script is so powerful. I’ve only dabbled so far and been impressed on how it can help me drill down the data for easier analysis on Google Sheets. Thanks for sharing these posts and guides on your website, Ben, and for your very informative newsletter which I always enjoy reading!
Hi! Sir Ben! I’m such a big fan of all your work. I just want to say thank you for keep sharing your idea to everyone <3
Wish you always in good health (bow)
Love your work, this was very easy to follow and a goood taster for what could be learned with some of the courses on offer here. Thanks again, this was great.
You’re welcome! Thanks for reading and good luck on your Apps Script journey!